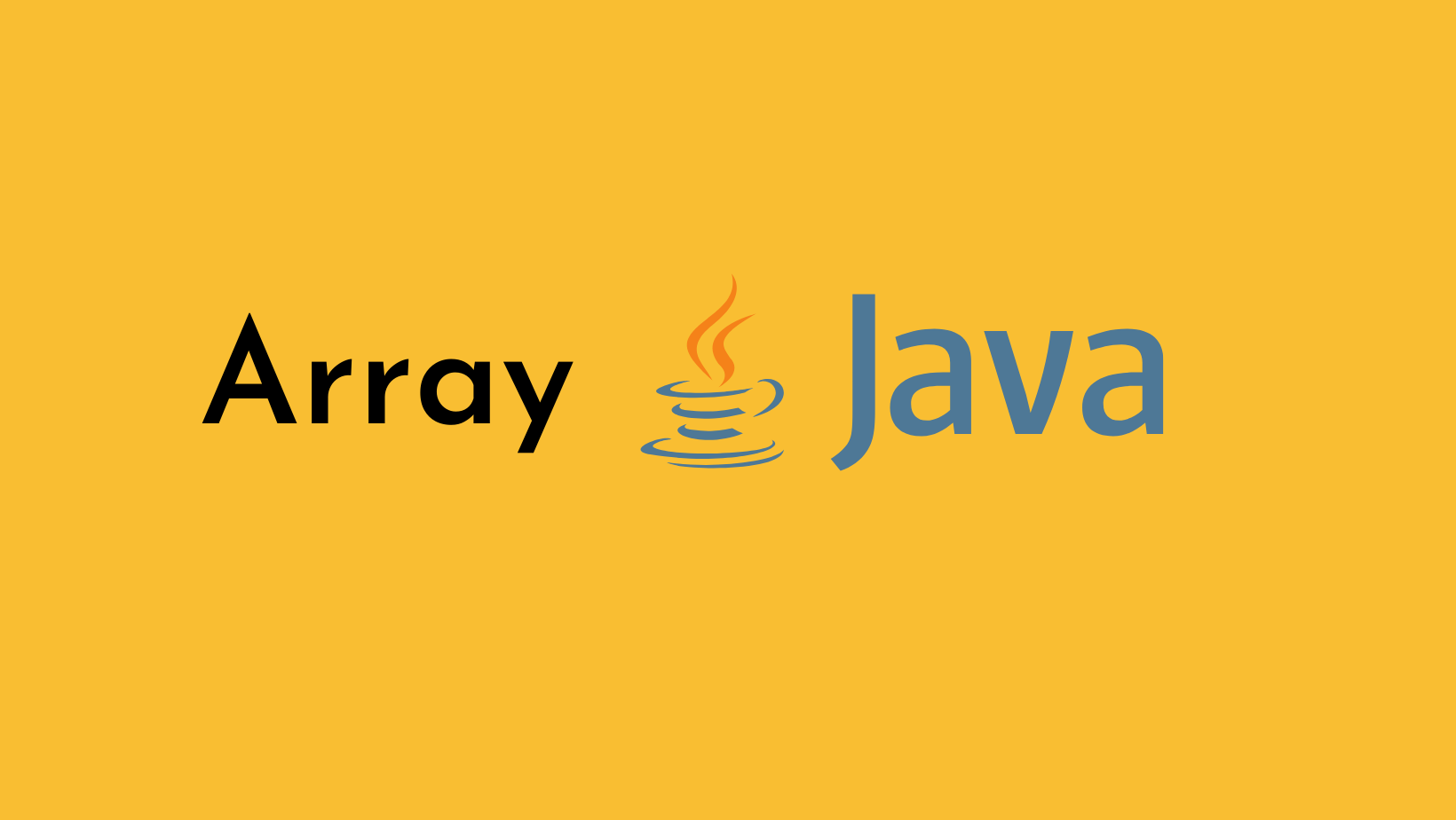
Data structure – Massiv/Array
In one of the previous blogs, we emphasized the importance of data structure. Data structures are ways of storing data, and many models are used in this process. Now let's take a look at the Array data structure.
Array is one of the most commonly used data structures. An array is a collection of elements of a fixed size that contains elements of a specified type (int, double, float, etc.).
Why array is used?
Storage of similar data types is often required during programming. To store this data, we need to define a variable equal to the number of data. But in this case it will be very difficult to remember the names of all the variables. Rather than naming all variables with a different name, a better method is to define an Array and store all elements in that Array.
How to create any array?
int[] num; // we defined the array
Above we defined an array of type int named num.
num = new int[10]; // we created the array
Above we have created an array of type int with a maximum number of elements of 10.
To create our array directly,
int[] num = new int[10];
Let's explain the above line:
int - the type of elements to be stored in the array
num - array name
10 - the maximum number of elements to be stored in the array
Let's take a look at the example below for better understanding. In this example, we want the output of the code to be the days of the week. Let's look at the coding of this issue. In one, we do not use an array, and in the other, we use an array to store data.
The difference is easy to see.
Let's also look at the main functions of the Array data structure:
Traverse − prints all elements of an array one by one.
Insertion − Inserts a new element at the given index.
Deletion − Deletes an element at the given index.
Search − Searches for an element using a given index or value.
Update− Updates the element at the given index.
So now you have basic information about Array/Array. You can use the following link for practical exercises.
https://bit.ly/3tQSppQ
Additionally, you can refer to the Fundamentals of Programming curriculum at ATL Academy for a deeper understanding of such data structure concepts and algorithms.